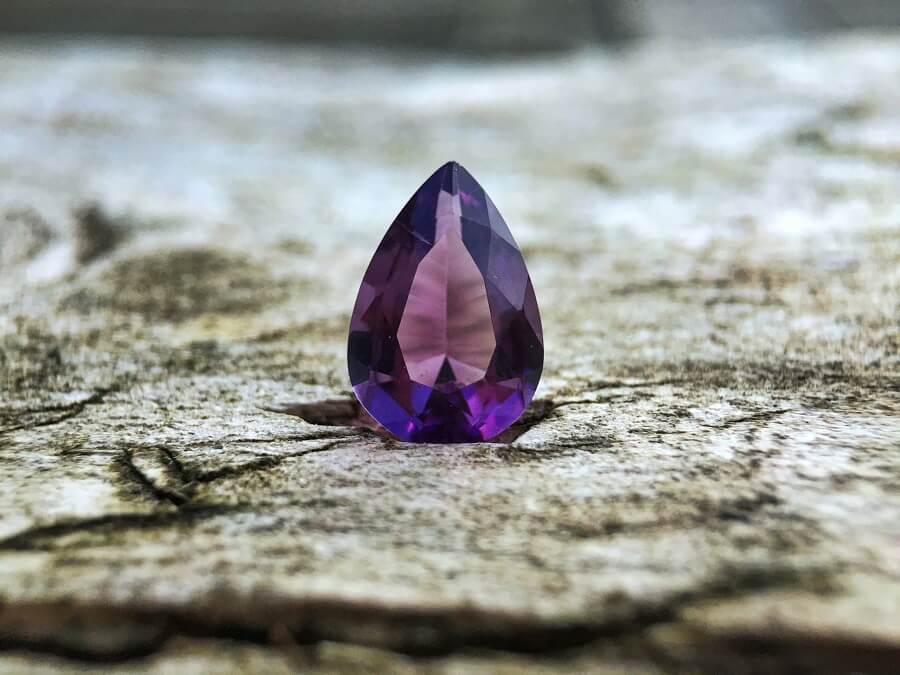
In the increasingly fast-paced world of software development, efficiency is a critical factor for success in the marketplace. Visual Studio, Microsoft’s flagship IDE (Integrated Development Environment), is packed full of features designed to make your programming experience as productive as possible. Among these is a lesser-known feature that is extremely useful; the ability to paste JSON from your clipboard as C# or Visual Basic classes.
This feature is a hidden gem for .NET developers working with JSON data, offering both a productivity boost and a reduction in the likelihood of manual coding errors. In this blog post, I will show you how to use the feature and I’ll also point out some alternatives if you need more advanced functionality.
JSON data modelling
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy for humans to read and write and is also efficient for machines to parse and generate. It is widely used in web development, APIs, and for configuring applications. Given its ubiquity, many developers are likely to be working with JSON data on an almost daily basis.
In addition to the aforementioned use cases, JSON can also be a good way to describe a data model that needs to be mapped to a database schema, given the ease of creating JSON snippets and quickly sharing these with a development team.
With this approach, it’s straightforward to see at a glance what the overall shape of the data model should be and to make quick adjustments as the structure is iterated on. When the data model is finalised, developers can map the JSON definition to entity classes when using an ORM (Object Relational Mapper), such as Entity Framework Core or Dapper.
Despite its simplicity and readability, manually mapping JSON data to classes can be tedious and error-prone. This is particularly true for complex JSON structures with nested objects and arrays. The risk of making mistakes increases with the complexity of the JSON, leading to potential bugs and wasted debugging time.
If only there was a better way… Oh wait, there is! Let’s check out how Visual Studio can alleviate the pain in the next section.
Enter the Paste JSON As Classes feature
Since Visual Studio 2019, it has been possible to automatically convert a JSON data structure into classes by simply copying and pasting a JSON string from your clipboard.
Let’s see how this works in the following subsections.
Copy the JSON
First, we need to copy some JSON to the clipboard.
If you want to do a quick test as you read this, you can copy the JSON found on the JSON Example page from json.org which I have reproduced below for your convenience.
{   "glossary": {     "title": "example glossary",     "GlossDiv": {       "title": "S",       "GlossList": {         "GlossEntry": {           "ID": "SGML",           "SortAs": "SGML",           "GlossTerm": "Standard Generalized Markup Language",           "Acronym": "SGML",           "Abbrev": "ISO 8879:1986",           "GlossDef": {             "para": "A meta-markup language, used to create markup languages such as DocBook.",             "GlossSeeAlso": [ "GML", "XML" ]           },           "GlossSee": "markup"         }       }     }   } }
The above JSON describes a Glossary data model with multiple nested objects.
Special pasting
Now that you have some JSON data on your clipboard, open Visual Studio if you haven’t already and then open an existing C# or Visual Basic project. Alternatively, you can create a new empty project of either type to test things out.
For the option to become available, make sure you have a .cs or .vb file opened and active within Visual Studio.
Next, access the following option from the main Visual Studio menu bar.
Edit –> Paste Special –> Paste JSON As Classes
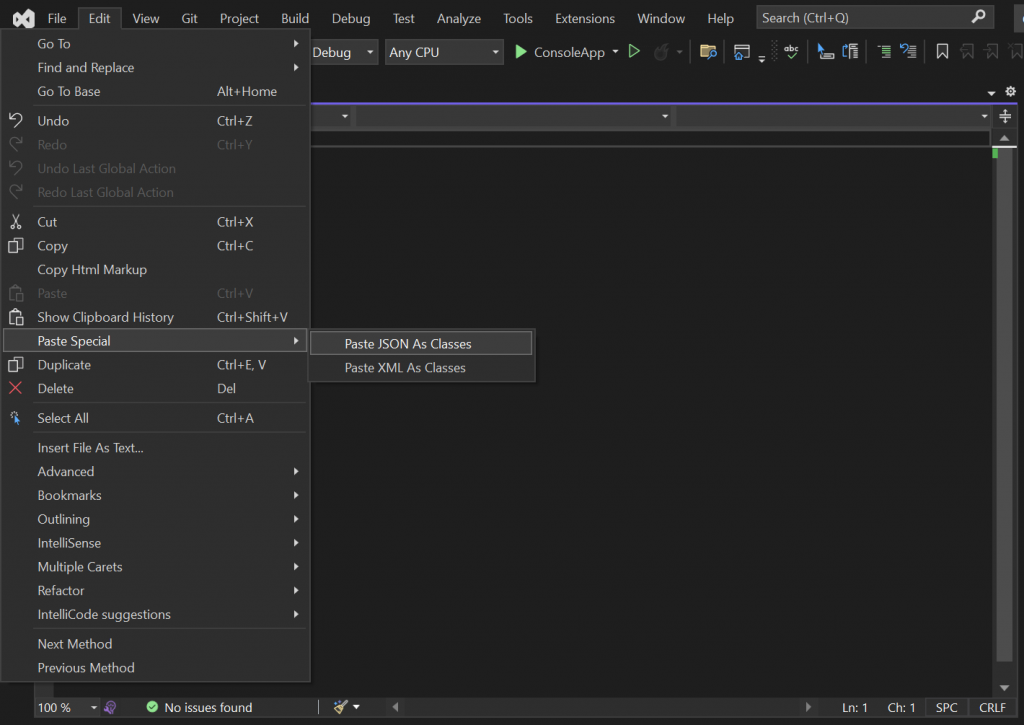
After selecting the ‘Paste JSON As Classes’ option, you should find that Visual Studio will convert the structure of the JSON string from your clipboard into separate classes for you, albeit within the same file.
Reviewing the results
The classes that are created for the Glossary JSON example should look like the following when pasting into a C# file.
public class Rootobject {     public Glossary glossary { get; set; } } public class Glossary {     public string title { get; set; }     public Glossdiv GlossDiv { get; set; } } public class Glossdiv {     public string title { get; set; }     public Glosslist GlossList { get; set; } } public class Glosslist {     public Glossentry GlossEntry { get; set; } } public class Glossentry {     public string ID { get; set; }     public string SortAs { get; set; }     public string GlossTerm { get; set; }     public string Acronym { get; set; }     public string Abbrev { get; set; }     public Glossdef GlossDef { get; set; }     public string GlossSee { get; set; } } public class Glossdef {     public string para { get; set; }     public string[] GlossSeeAlso { get; set; } }
That’s pretty neat! You can imagine how much time this can save when working with a large JSON data model.
Even though all the classes are in a single file, it is straightforward to move them into separate files using the Visual Studio refactoring tools, via the Ctrl + . shortcut and the ‘Move type to’ option.
Regarding naming conventions, you’ll notice that Visual Studio has matched the names of the C# classes and properties exactly according to what was defined in the JSON. So in this example, there is a mixture of camel casing and Pascal casing. Unfortunately, there aren’t any options for controlling the naming conventions when pasting the JSON, but I think this is a relatively minor inconvenience when thinking about the time the feature already saves.
On another note, if you happen to be using Visual Basic, the output will look as follows.
Public Class Rootobject     Public Property glossary As Glossary End Class Public Class Glossary     Public Property title As String     Public Property GlossDiv As Glossdiv End Class Public Class Glossdiv     Public Property title As String     Public Property GlossList As Glosslist End Class Public Class Glosslist     Public Property GlossEntry As Glossentry End Class Public Class Glossentry     Public Property ID As String     Public Property SortAs As String     Public Property GlossTerm As String     Public Property Acronym As String     Public Property Abbrev As String     Public Property GlossDef As Glossdef     Public Property GlossSee As String End Class Public Class Glossdef     Public Property para As String     Public Property GlossSeeAlso() As String End Class
Another thing to be aware of is the ability to paste XML as classes. You may have already noticed the ‘Paste XML As Classes’ option that is available when selecting Edit –> Paste Special in Visual Studio. Pasting XML as classes can be useful for many of the same reasons that pasting JSON as classes is useful, especially if you need to work with legacy XML-based APIs for which there may not be a wrapper library.
Alternatives
While the ‘Paste JSON As Classes’ feature in Visual Studio is very convenient, it doesn’t offer any customisability.
If you want more control over how the JSON is converted to classes, you’ll need to turn elsewhere.
Let’s take a look at a couple of alternatives.
JSON2CSharp
JSON2CSharp is an awesome online tool for converting JSON to C# and has lots of different settings to choose from before you process the conversion. This includes everything from using Pascal Case to controlling whether JsonProperty
attributes should be added to the properties (or fields).
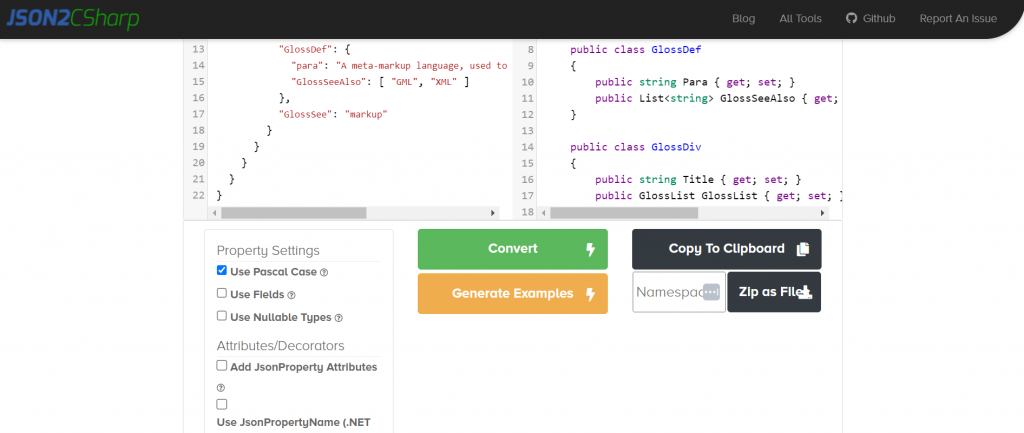
If you don’t have any issues with pasting the JSON you need to convert into an online tool and there isn’t any sensitive data in your JSON payload, I highly recommend that you check it out.
quicktype
quicktype is another great online tool, with an equally impressive range of customisation options to choose from.
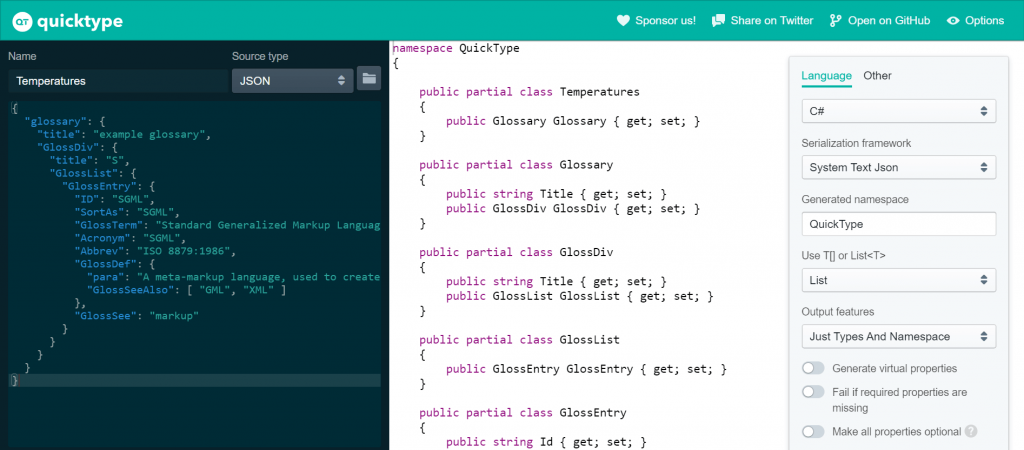
Although there isn’t an option to choose between camel case and Pascal case, the code is output using Pascal case to match the usual C# naming conventions, which is probably what you will want anyway.
There is also a Visual Studio quicktype plugin available!
Summary
The ‘Paste JSON As Classes’ option within Visual Studio is a very helpful yet underutilized feature.
Some may argue that it is not as useful as it could be, seeing that it lacks customisation options such as the configuration of camel vs Pascal casing. However, I still find the feature to be valuable, given the convenience of having it built directly into the IDE and the enhanced privacy compared to pasting data into an online service that may offer more functionality.
I hope that if you weren’t aware of this feature already, you will remember in the future that it is available and that you will find it to be an effective means of streamlining the conversion of JSON data into classes within your codebase.
Comments